Improving React Performance: Best Practices for Optimization
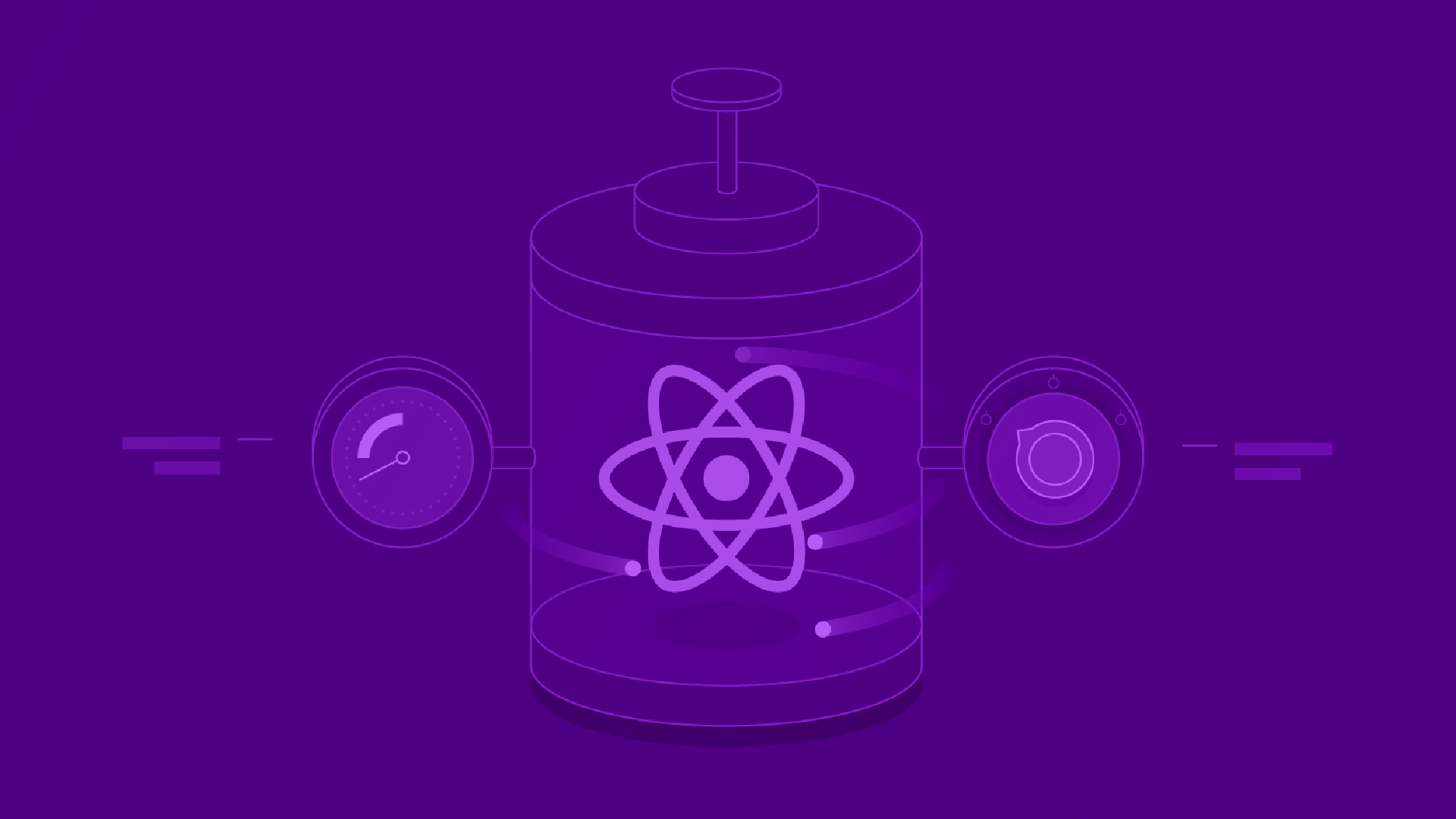
MERN—the magical acronym that encapsulates the power of MongoDB, Express.js, React, and Node.js—beckons full-stack developers into its realm. Our focus for this blog will be on React, the JavaScript library that’s revolutionized user interfaces on the web.
What is React?
React is a JavaScript library for creating dynamic and interactive user interfaces. Since its inception, react has gained immense popularity and has become one of the leading choices among developers for building user interfaces. It has become the go-to library for building user interfaces, thanks to its simplicity and flexibility. However, as applications grow in complexity, React’s performance can become a concern. Slow-loading pages and unresponsive user interfaces can lead to poor user experience. Fortunately, there are several best practices and optimization techniques that can help you improve React performance.
Ignitho has been developing enterprise full stack apps using react for the last many years. We also recently had a tech talk on “Introduction to React”. In this blog post, which is the first part of MERN, we will be discussing React and explore some of the strategies to ensure your React applications run smoothly.
- Use React’s Built-in Performance Tools
React provides a set of built-in tools that can help you identify and resolve performance bottlenecks in your application. The React Dev Tools extension for browsers, for instance, allows you to inspect the component hierarchy, track component updates, and analyze render times. By using these tools, you can gain valuable insights into your application’s performance and make targeted optimizations.
- Functional Components & Component Interaction
The subtle way of optimizing performance of React applications is by using functional components. Though it sounds cliche, it is the most straightforward and proven tactic to build efficient and performant React applications speedily.
Experienced React developers suggest keeping your components small because smaller components are easier to read, test, maintain, and reuse.
Some advantages of using React components are:
- Makes code more readable
- Easy to test
- Yields better performance
- Debugging is a piece of cake, and
- Reducing Coupling Factor
- Optimize Rendering with Pure Component and React.memo
React offers two ways to optimize rendering: Pure Component and React.memo.
- Pure Component: This is a class component that automatically implements the should Component Update method by performing a shallow comparison of props and state. Use it when you want to prevent unnecessary renders in class components.
class MyComponent extends React.PureComponent {
// …
}
- React.memo: This is a higher-order component for functional components that memoizes the component’s output based on its props. It can significantly reduce re-renders when used correctly.
const MyComponent = React.memo(function MyComponent(props) {
// …
});
By using these optimizations, you can prevent unnecessary renders and improve your application’s performance.
- Memoize Expensive Computations
Avoid recalculating values or making expensive computations within render methods. Instead, memoize these values using tools like useMemo or useSelector (in the case of Redux) to prevent unnecessary work during renders.
const memoizedValue = useMemo(() => computeExpensiveValue(dep1, dep2), [dep1, dep2]);
- Avoid Reconciliation Pitfalls
React’s reconciliation algorithm is efficient, but it can still lead to performance issues if not used wisely. Avoid using array indices as keys for your components, as this can cause unnecessary re-renders when items are added or removed from the array. Instead, use stable unique identifiers as keys.
{items.map((item) => (
<MyComponent key={item.id} item={item} />
))}
Additionally, be cautious when using setState in a loop, as it can trigger multiple renders. To batch updates, you can use the functional form of setState.
this.setState((prevState) => ({
count: prevState.count + 1,
}));
- Lazy Load Components
If your application contains large components that are not immediately visible to the user, consider lazy loading them. React’s React.lazy() and Suspense features allow you to load components asynchronously when they are needed. This can significantly improve the initial load time of your application.
const LazyComponent = React.lazy(() => import(‘./LazyComponent’));
function MyComponent() {
return (
<div>
<Suspense fallback={<LoadingSpinner />}>
<LazyComponent />
</Suspense> </div>
);
}
- Profile and Optimize Components
React provides a built-in profiler that allows you to analyze the performance of individual components. By using the React.profiler API, you can identify components that are causing performance bottlenecks and optimize them accordingly.
import { unstable_trace as trace } from ‘scheduler/tracing’;
function MyComponent() {
trace(‘MyComponent render’);
// …
}
- Bundle Splitting
If your React application is large, consider using code splitting to break it into smaller, more manageable chunks. Tools like Webpack can help you achieve this by generating separate bundles for different parts of your application. This allows for faster initial load times, as users only download the code they need.
- Use PureComponent for Lists
When rendering lists of items, use React.PureComponent or React.memo for list items to prevent unnecessary re-renders of list items that haven’t changed.
function MyList({ items }) {
return (
<ul>
{items.map(item => (
<MyListItem key={item.id} item={item} />
))}
</ul>
);
}
const MyListItem = React.memo(function MyListItem({ item }) {
// …
});
- Optimize Network Requests
Efficiently handling network requests can have a significant impact on your application’s performance. Use techniques like caching, request deduplication, and lazy loading of data to minimize the network overhead.
- Regularly Update Dependencies
Make sure to keep your React and related libraries up to date. New releases often come with performance improvements and bug fixes that can benefit your application.
- Trim JavaScript Bundles
If you wish to eliminate code redundancy, learn to trim your JavaScript packages. When you cut-off duplicates and unnecessary code, the possibility of your React app performance multiplies. You must analyze and determine bundled code.
- Server-Side Rendering (SSR)
NextJS is the best among the available SSR. It is getting popular amongst developers & so is the usage of the NextJS-based React Admin Dashboard. NextJS integrated React admin templates can help you boost the development process with ease.
In conclusion, improving React performance is essential for delivering a smooth user experience. By following these best practices and optimization techniques, you can ensure that your React applications remain fast and responsive, even as they grow in complexity. Remember to profile your application, measure the impact of optimizations, and stay up to date with the latest developments in the React ecosystem to keep your application running at its best.
In the next blog we will be covering MongoDB. Get in touch with our Product Engineering tribe for the latest around MERN.